Just create the simple MVC Application and you have structure
Like Below screen;
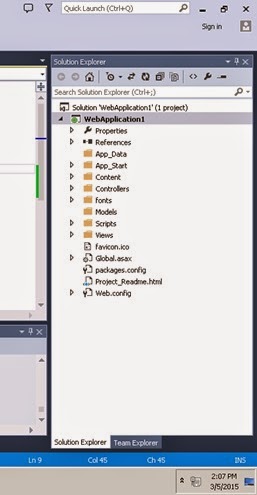
Now you can see that we don’t have Models file in the
structure. Now Create a Department class and the model which will have 3 Entity
:
DepartmentID, Name, NumberoFMember
DepartmentID, Name, NumberoFMember
namespace WebApplication1.Models
{
public class Department
{
public int DepartmentID { get; set; }
public string Name { get; set; }
public int NumberOfMember { get; set; }
}
}
Now create the Controller and Create the View to display
that model into View Side;
Now created Controller like Below;
namespace WebApplication1.Controllers
{
public class DepartmentDisplayController : Controller
{
//
// GET:
/DepartmentDisplay/
public ActionResult Index()
{
return View();
}
}
}
Take the reference of the department into the Controller;
using WebApplication1.Models;
Now assign the object to the department class;
public ActionResult Index()
{
Department dpt = new Department();
dpt.DepartmentID = 1234;
dpt.Name = "IT";
dpt.NumberOfMember = 3;
return View();
}
Now you need to return the created object through View
return View(dpt);
Create the view as strongly type like below; Right Click
on ActionResult of Index();
Now give the Name to the AddView with the name as Index;
Giving View Name : Index; and Select the Model Class name as Department.
Click on Add; Then it will create the View Class like below;
Open the Index.cshtml;
Put the code to see
the Value that is in model;
@model
WebApplication1.Models.Department
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div>
Department ID : <b> @Html.Label(Model.DepartmentID.ToString())</b><br />
Department Name :<b> @Html.Label(Model.Name.ToString())</b><br />
Department Member :<b> @Html.Label(Model.NumberOfMember.ToString())</b><br />
</div>
</body>
</html>
We can see the Output as;
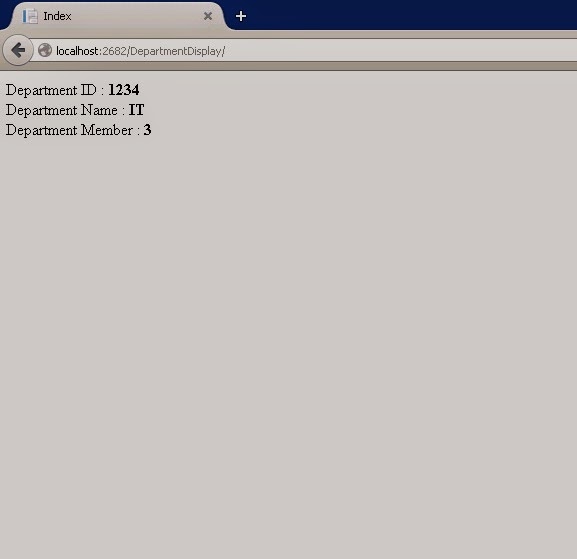
No comments:
Post a Comment